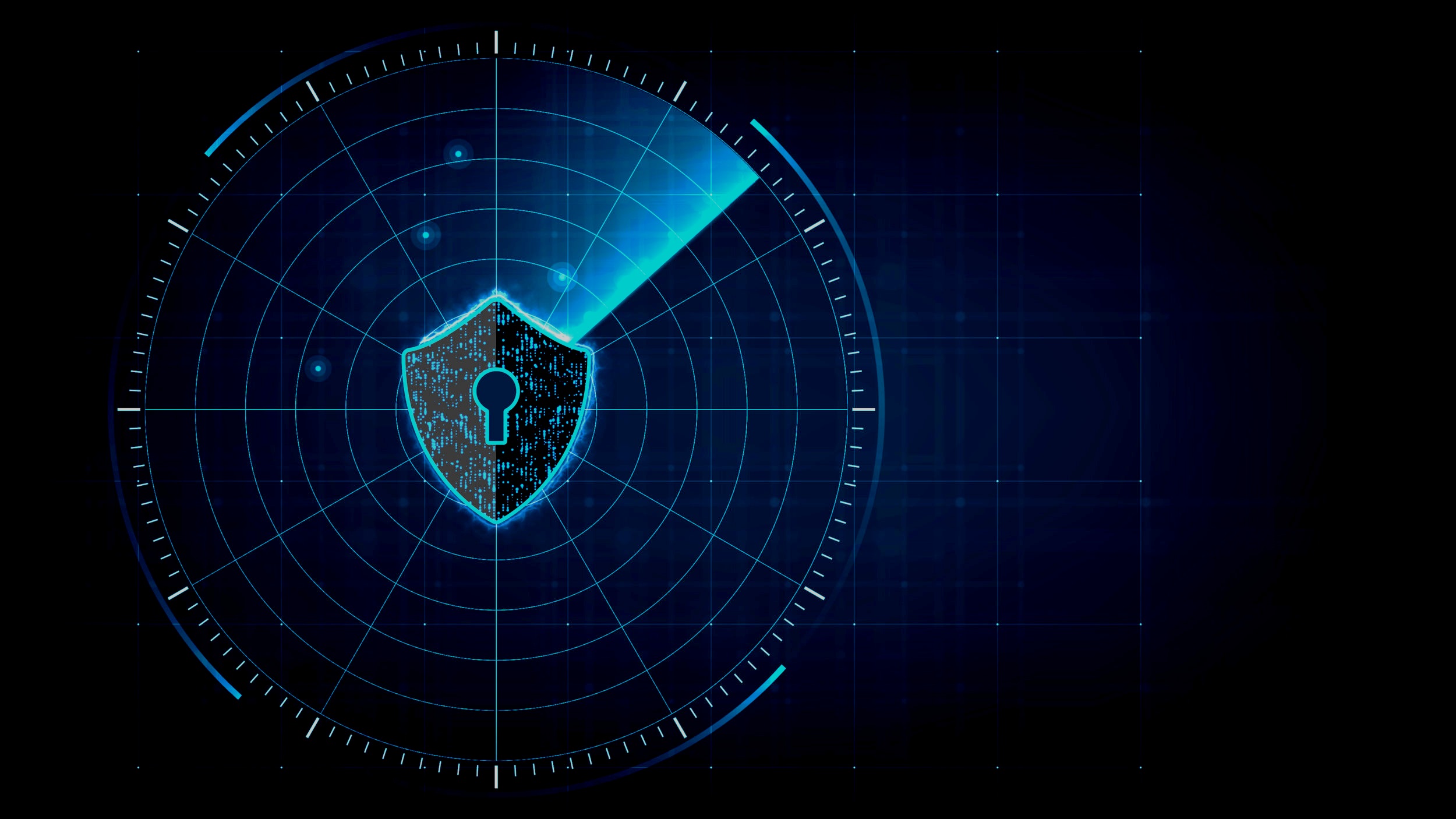
What is a vulnerability scanning ?
Vulnerability scanning is an automated process that allows the organizations to check if their networks, systems and applications have security vulnerabilities that could expose them to attacks. Vulnerability scanning is performed by the IT department of the organization or a third-party security service provider. This scan is also performed by the attackers who try to discover entry points into the target network.
How does vulnerability scanner work ?
A vulnerability scanner uses a database that contains known vulnerabilities, coding bugs, packet construction anomalies, default configurations, and potential paths to sensitive data that can be exploited by attackers. The vulnerability scanner scans the target then compares the results to the database. After that the vulnerability scanner provides a report with findings that can be analyzed to improve the security posture of the oraganization.
In this article, we are going to build a vulnerability scanner based on the port scanner script that we are implemented in the previous article. So, we are going to convert the port scanner program into a class then import it into our vulnerability scanner program to scan our target then determine the open ports and discover some of services that are running over these open ports then we are going to create a list of common vulnerable services in a text file as our database to compare our discovered services with that list and if we find matches that means we discovered vulnerable services that can be exploited.
Converting port scanner into a class
Here is a port scanner class as depicted below. what we just did is defining the class name “class portscan()” at the first, also we defined two lists “banners” and “open_ports” that we will use to store the discovered open ports and banners. Then we defined the “init()” function that a reserved function in python with two parameters or attributes “target” and “port_num”. This function initializes the attributes of the obiects that will be created of this class. The “self” represents the instance or the object of the class. We use “self” keyword to access the attributes and methods of the class in python. After that, we modified the scan() function by placing the converted_ip variable into scan_port() function instead of scan() function and removing the print statement as we do not need it anymore. Also, we removed the get_banner() function and replaced it by defining a banner variable at the scan_port() function.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
import socket
from IPy import IP
class portscan():
banners = []
open_ports = []
def __init__(self, target, port_num):
self.target = target
self.port_num = port_num
def scan(self):
for port in range(1, self.port_num):
self.scan_port(port)
def check_ip(self):
try:
IP(self.target)
return(self.target)
except ValueError:
return socket.gethostbyname(self.target)
def scan_port(self, port):
try:
converted_ip = self.check_ip()
sock = socket.socket()
sock.settimeout(0.5)
sock.connect((converted_ip, port))
self.open_ports.append(port)
try:
banner = sock.recv(1024).decode().strip('\n').strip('\r')
self.banners.append(banner)
except:
self.banners.append(' ')
sock.close()
except:
pass
Writing the vulnerability scanner script
Step 1: Importing port scanner class
We import the port scanner class that contains all the required libraries, variables, and functions.
1
2
# Python code snippet
import portscanner
Step 2: Asking user for input
We ask a user to enter the target_ip, port_number, and vul_file that contain the common vulnerabilities list.
1
2
3
4
5
# Python code snippet
targets_ip = input('[+] * Enter Target To Scan For Vulnerable Open Ports: ')
port_number = int(input('[+] * Enter Amount Of Ports You Want To Scan (500 - First 500 Ports): '))
vul_file = input('[+] * Enter Path To The File With Vulnerable Softwares: ')
print('\n')
Step 3: Discovering the vulnerable ports
At the first, we define a port scanner object “target” and pass the two parameters that the user specified them “targets_ip” and “port_number” to initialize them at the port scanner constructor “init()”. Then we call the scan() function that calls all the other functions of the port scanner class on our defined object “target”. After that, we open the “vul_file” with a read option ‘r’. Also we create the count variable to keep track of the banners with the corresponding open ports and increase it after each new banner selected. Then we implement a nested for loop to iterate over the banners list and compare each banner with each line of the vul_file. If the banner is existed in the vul_file, then we have found a vulnerable service and print it with the corresponded port. We use “file.seek(0)” to set the pointer at the first line of the vul_file at each time we select a new banner.
1
2
3
4
5
6
7
8
9
10
11
#Python code snippet
target = portscanner.portscan(targets_ip, port_number)
target.scan()
with open(vul_file,'r') as file:
count = 0
for banner in target.banners:
file.seek(0)
for line in file.readlines():
if line.strip() in banner:
print('[!!] VULNERABLE BANNER: "' + banner + '" ON PORT: ' + str(target.open_ports[count]))
count += 1
The complete vulnerability scanner script
Here is a final vulnerability scanner script as depicted below.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
import portscanner
targets_ip = input('[+] * Enter Target To Scan For Vulnerable Open Ports: ')
port_number = int(input('[+] * Enter Amount Of Ports You Want To Scan (500 - First 500 Ports): '))
vul_file = input('[+] * Enter Path To The File With Vulnerable Softwares: ')
print('\n')
target = portscanner.portscan(targets_ip, port_number)
target.scan()
with open(vul_file,'r') as file:
count = 0
for banner in target.banners:
file.seek(0)
for line in file.readlines():
if line.strip() in banner:
print('[!!] VULNERABLE BANNER: "' + banner + '" ON PORT: ' + str(target.open_ports[count]))
count += 1
Script output
We test our script in the virualbox environment that consisted of two virtual machines (Kali Linux and Metasploitable). We run the script in the kali linux machine to scan our target which is the metasploitable machine. And here is our script results.
As depicted in the image above, we successfully discovered two vulnerable services are running over the metasploitable machine.
Note: Keep the vulnerability scanner script, port scanner class, and the vulnerable banners text file in the same directory/folder.